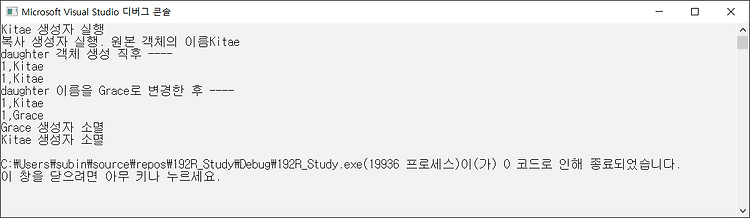
[C++] 명품 C++ 프로그래밍 예제 05-11 분석
두비니
·2019. 12. 5. 02:40
[C++] 명품 C++ 프로그래밍 예제 05-11 분석
//05_10에서 깊은복사까지 추가한 코드임
#include <iostream>
#include <cstring>
using namespace std;
class Person {
char* name;
int id;
public:
Person(int id, const char* name);
Person(Person& p);
~Person();
void changeName(const char *name);
void show() { cout << id << ',' << name << endl; }
};
Person::Person(Person& p) {
this->id = p.id;
int len = strlen(p.name);
this->name = new char[len+1];
strcpy(this->name, p.name);
cout << "복사 생성자 실행. 원본 객체의 이름" << this->name << endl;
}
Person::Person(int id, const char* name) {
this->id = id;
int len = strlen(name);
this->name = new char[len + 1];
//이름을 바로 복사하는게 아니라 일단 공간을 할당하고, 그 뒤에 복사해주기
strcpy(this->name, name);
cout <<this->name << " 생성자 실행" << endl;
}
void Person::changeName(const char* name) {
/*if (strlen(name) > strlen(this->name))
return;*/
//이거는 메모리 문제때문에 아예 막아놓음
if (this->name) {
delete[] this->name;
this->name = NULL;
}
this->name = new char[strlen(name) + 1];
strcpy(this->name, name);
}
Person::~Person() {
cout << this->name << " 생성자 소멸" << endl;
if (name) {
delete[] name;
name = NULL;
}
}
int main() {
Person father(1, "Kitae"); // (1) father 객체 생성
Person daughter(father); // (2) daughter 객체 복사 생성. 복사생성자호출
cout << "daughter 객체 생성 직후 ----" << endl;
father.show(); // (3) father 객체 출력
daughter.show(); // (3) daughter 객체 출력
daughter.changeName("Grace"); // (4) daughter의 이름을 "Grace"로 변경
cout << "daughter 이름을 Grace로 변경한 후 ----" << endl;
father.show(); // (5) father 객체 출력
daughter.show(); // (5) daughter 객체 출력
return 0; // (6), (7) daughter, father 객체 소멸
}
다음 코드는 예제 5-11에서 추가적으로 더 잘 파악하기 위해 몇가지 코드를 더 추가한 모습입니다.
다른점이 있다면 교재의 changeName은 주석부분처럼 더 길면 복사자체가 불가능하게 해놓았지만, 저는 코드를 길이에 상관없게 실행되게끔 하였습니다.
보면 잘 실행되는것 볼 수 있죠.
봐야 할 점은 복사생성자 실행시에 복사생성자가 따로 실행된다는점,
따로 사용자가 복사생성자를 선언해주었기 때문에 메모리 중복이 생기지 않는다는 점,
그리고 메모리는 LIFO구조로 관리되기때문에 나중에 생성된 Grace부터 소멸이 진행된다는 점.
봐두면 좋을 것 같습니다.ㅎ.ㅎ.ㅎ....ㅎ.
보면 5글자 이상으로 바꿔도 상관없이 짠 걸 알 수 있습니다.
'Coding_Algorithm > C_C++' 카테고리의 다른 글
[C] pthread_mutex 이해하기 (0) | 2021.05.07 |
---|---|
[C/C++] sprintf, snprintf (0) | 2021.03.04 |
[C언어] C언어로 수박받기 게임을 만들어보았습니다. (1) | 2020.08.17 |
[재귀함수] 재귀함수 구현할 때 염두에 두면 좋은 것들 (0) | 2020.05.02 |
[C++] 묵시적 복사 생성 (0) | 2019.12.05 |